Golang
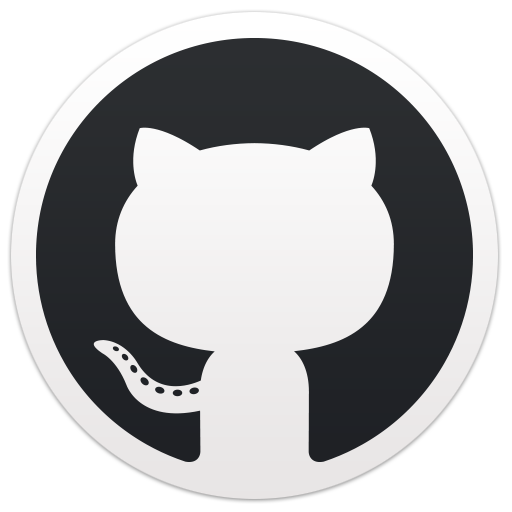
Installation
If you are using golang modules make sure you
include the /v4
at the end of your import paths
$ go get github.com/mailgun/mailgun-go/v4
If you are not using golang modules, you can drop the /v4
at the end of the import path.
As long as you are using the latest 1.10 or 1.11 golang release, import paths that end in /v4
in your code should work fine even if you do not have golang modules enabled for your project.
$ go get github.com/mailgun/mailgun-go
Usage
Here's a simple example on how to send an email. As always, please consult the repository readme for full details.
package main
import (
"context"
"fmt"
"log"
"time"
"github.com/mailgun/mailgun-go/v4"
)
// Your available domain names can be found here:
// (https://app.mailgun.com/app/domains)
var yourDomain string = "your-domain-name" // e.g. mg.yourcompany.com
// You can find the Private API Key in your Account Menu, under "Settings":
// (https://app.mailgun.com/app/account/security)
var privateAPIKey string = "your-private-key"
func main() {
// Create an instance of the Mailgun Client
mg := mailgun.NewMailgun(yourDomain, privateAPIKey)
//When you have an EU-domain, you must specify the endpoint:
//mg.SetAPIBase("https://api.eu.mailgun.net/v3")
sender := "sender@example.com"
subject := "Fancy subject!"
body := "Hello from Mailgun Go!"
recipient := "recipient@example.com"
// The message object allows you to add attachments and Bcc recipients
message := mg.NewMessage(sender, subject, body, recipient)
ctx, cancel := context.WithTimeout(context.Background(), time.Second*10)
defer cancel()
// Send the message with a 10 second timeout
resp, id, err := mg.Send(ctx, message)
if err != nil {
log.Fatal(err)
}
fmt.Printf("ID: %s Resp: %s\n", id, resp)
}